How To Execute Rest API
This page explains in detail how to execute Rest API's exposed by Continuous Testing.
Demonstration about how to get application information and upload an application to Cloud is described below.
Rest API Authorization
These are important keys for the examples described below:
- YOUR_ACCESS_KEY - A security token to execute Rest API. To obtain your Access see Obtain Your Access Key.
- CLOUD_SERVER - Cloud Server URL
Cloud Server requires authentication and authorization to perform REST Operations. The authentication can be done in the following ways:
If Two-Factor Authentication (2FA) is in use, only Access Key authentication works.
Bearer Token - Access Key
Postman
Use Username and Password
Postman
**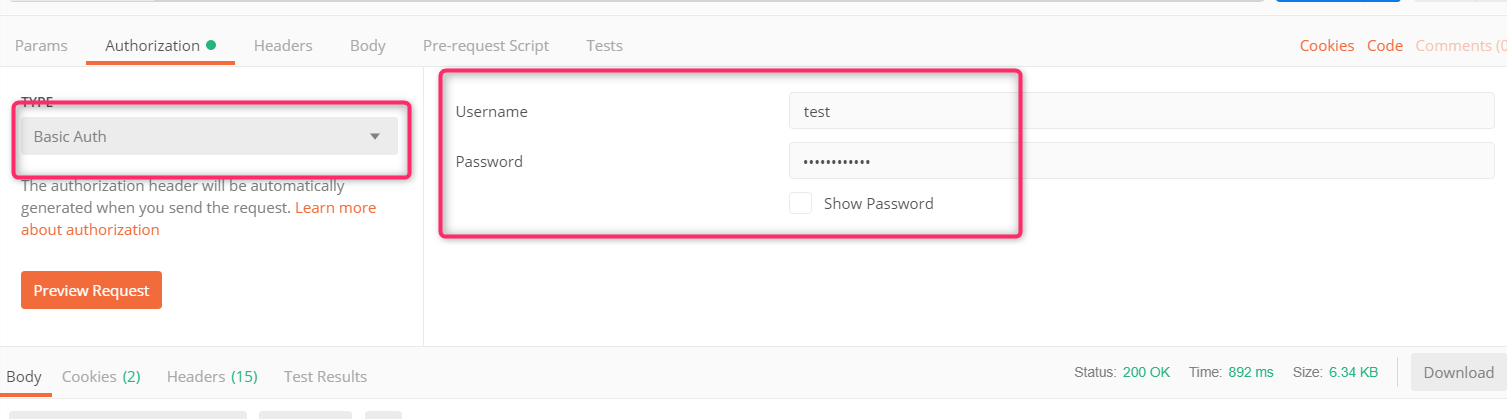
**
An authenticated user takes a specific role and perform the operation. Typically the role is assigned at the time when a user is created.
Example - Get Application Information (GET)
The Get Applications Rest API allows Project Administrators to get applications and is defined as GET/API/v1/applications.
The GET requests for Applications supports filtering.
Filter Syntax
GET URL?filtername=value
For example, in GET /API/v1/applications?osType=android, "osType" is a filter name and "android" is the value. This request returns all android applications.
Use CURL
CURL is commonly used to transfer data across different underlying protocols. It is also popularly used to perform RESToperations.
Here is an example for Get Applications Rest API.
Get Applications Using CURL
>>>curl -H "Authorization: Bearer YOUR_ACCESS_KEY" CLOUD_SERVER/api/v1/applications?"osType=android&packageName=com.experitest.ExperiBank"
HTTP/1.1 200
.
.
[ {
"id" : 4924493,
"name" : "com.experitest.ExperiBank/.LoginActivity",
"packageName" : "com.experitest.ExperiBank",
"version" : "1.0",
"releaseVersion" : "1",
"applicationName" : "EriBank",
"uniqueName" : null,
"notes" : null,
"cameraSupport" : false,
"osType" : "ANDROID",
"createdAt" : 1550850592658,
"createdAtFormatted" : "2019-02-22 17:49:52",
"mainActivity" : ".LoginActivity",
"productId" : null,
"bundleIdentifier" : null,
"instrumentByProfile" : null,
"nonInstrumented" : false,
"hasCustomKeystore" : false,
"isForSimulator" : false,
"projectsInfo" : null,
"canDelete" : true
} ]
In this command, ?osType=android&packageName=com.experitest.ExperiBank is the filter syntax. osType and packageName are filters and are used to filter the applications.
Use POSTMAN
Postman is an application which makes API development faster and easy. It is installed in Chome.
This screenshot shows how to execute Get Applications using a filter.
Use JAVA
Gradle Dependency Expand source
dependencies {
compile group: 'com.mashape.unirest', name: 'unirest-java', version: '1.4.9'
}
Code Example - Get Application Expand source
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.JsonNode;
import com.mashape.unirest.http.Unirest;
import com.mashape.unirest.http.exceptions.UnirestException;
import org.apache.http.HttpHeaders;
import org.testng.annotations.*;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Objects;
public class RestProjectAPI {
private HttpResponse<String> responseString;
private HttpResponse<InputStream> responseInputStream;
private String urlBase = "http://hostname:port/"; //TODO: modify hostname and port of your Reporter
private String user = "user"; //TODO: user name
private String password = "....."; //TODO: user password
private String accessKey= "....."; //TODO: user access key
String projectName = "projectName";//TODO: project name is here
String projectID = "projectID";//TODO: project ID is here
@Test
public void getApplications() {
String url = urlBase + "/api/v1/applications?osType=android&packageName=com.experitest.ExperiBank";
try {
responseString = Unirest.get(url)
.basicAuth(user, password)
.header("content-type", "application/json")
.asString();
System.out.println(responseString.getBody());
} catch (Exception e) {
e.printStackTrace();
}
}
Example - Upload an Application (POST)
This Rest API uploads an application and gets the application ID.
This is a POST request and defined as POST /API/v1/applications/new.
Use CURL
Because this API is a POST request, it can contain a parameter which can be sent as headers as well as in the body. Typically in CURL commands, header parameters are sent with -H parameter and body parameters are sent with -F parameter.
Upload Application using CURL
>> curl -v -i -X POST -k -H "Authorization: Bearer YOUR_ACCESS_KEY" -F "uniqueName=UICatalog" -F file=@C:\com.experitest.uicatalog_.MainActivity_ver_3.1710.apk CLOUD_SERVER/api/v1/applications/new
HTTP/1.1 200
{"status":"SUCCESS","data":{"created":"true","id":"5754383"},"code":"OK"}*
- In the above command "Authorization" is passed as a header because its send with -H option and "uniqueName" and "file" are sent as a body since it is sent as -F.
- The file parameter value i.e file=@ has a character '@', this indicates that the file is uploaded as form based.
Use POSTMAN
This screenshot shows the usage of the Rest API.
Note how file and uniquename are sent as the body.
Using JAVA
Gradle Dependency Expand source
dependencies {
compile group: 'com.mashape.unirest', name: 'unirest-java', version: '1.4.9'
}
Code Example - Upload Application Expand source
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.JsonNode;
import com.mashape.unirest.http.Unirest;
import com.mashape.unirest.http.exceptions.UnirestException;
import org.apache.http.HttpHeaders;
import org.testng.annotations.*;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Objects;
public class RestProjectAPI {
private HttpResponse<String> responseString;
private HttpResponse<InputStream> responseInputStream;
private String urlBase = "http://hostname:port/"; //TODO: modify hostname and port of your Reporter
private String user = "user"; //TODO: user name
private String password = "....."; //TODO: user password
private String accessKey= "....."; //TODO: user access key
String projectName = "projectName";//TODO: project name is here
String projectID = "projectID";//TODO: project ID is here
@Test
public void uploadApp() {
String url = urlBase + "/api/v1/applications/new";
try {
Unirest.post(url)
.basicAuth(user, password)
.field("file", new File("C:\\Users\\TestUser\\Downloads\\com.experitest.uicatalog_.MainActivity_ver_3.1710.apk"))
.asBinary();
} catch (Exception e) {
e.printStackTrace();
}
}
}